Ferris on LCD Display
Let's create Ferris (my attempt to make it look like a crab; if you have a better design, feel free to send a pull request) using a single character. In fact, we can combine 4 or 6 adjacent grids to display a single symbol. Creativity is up to you, and you can improve it however you like.
We'll use the custom character generator from the previous page to create this symbol. This will give us the byte array that we can use.
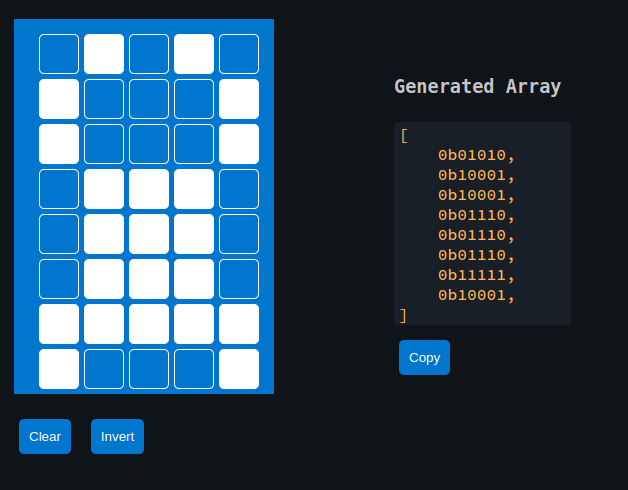
Note that the previous crate hd44780-driver doesn't support custom characters. To handle this, we can use the liquid_crystal crate, which allows us to work with custom characters.
Initialize the LCD interface
#![allow(unused)] fn main() { let mut lcd_interface = Parallel::new(d4, d5, d6, d7, rs, en, lcd_dummy); let mut lcd = LiquidCrystal::new(&mut lcd_interface, Bus4Bits, LCD16X2); lcd.begin(&mut timer); }
Our generated byte array for the custom character
#![allow(unused)] fn main() { const FERRIS: [u8; 8] = [ 0b01010, 0b10001, 0b10001, 0b01110, 0b01110, 0b01110, 0b11111, 0b10001, ]; // Define the character lcd.custom_char(&mut timer, &FERRIS, 0); }
Displaying
Displaying the character is straightforward. You just need to use the CustomChar enum and pass the index of the custom character. We've defined only one custom character, which is at position 0.
#![allow(unused)] fn main() { lcd.write(&mut timer, CustomChar(0)); lcd.write(&mut timer, Text(" implRust!")); }
Clone the existing project
You can clone (or refer) project I created and navigate to the lcd-custom
folder.
git clone https://github.com/ImplFerris/pico2-rp-projects
cd pico2-projects/lcd-custom/