Multi Custom character
I attempted to create the Ferris image using 6 adjacent grids with the generator from the previous page. Here’s the Rust code to utilize those byte arrays.
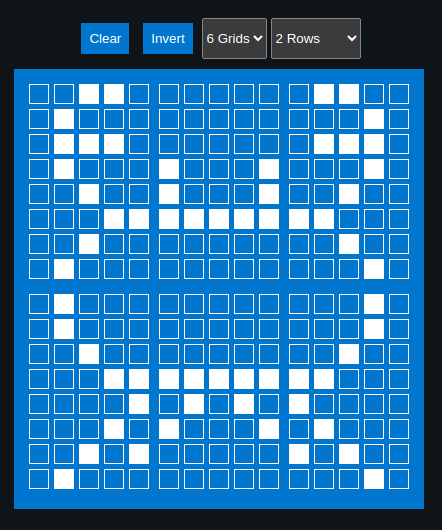
Generated Byte array for the characters
#![allow(unused)] fn main() { const SYMBOL1: [u8; 8] = [ 0b00110, 0b01000, 0b01110, 0b01000, 0b00100, 0b00011, 0b00100, 0b01000, ]; const SYMBOL2: [u8; 8] = [ 0b00000, 0b00000, 0b00000, 0b10001, 0b10001, 0b11111, 0b00000, 0b00000, ]; const SYMBOL3: [u8; 8] = [ 0b01100, 0b00010, 0b01110, 0b00010, 0b00100, 0b11000, 0b00100, 0b00010, ]; const SYMBOL4: [u8; 8] = [ 0b01000, 0b01000, 0b00100, 0b00011, 0b00001, 0b00010, 0b00101, 0b01000, ]; const SYMBOL5: [u8; 8] = [ 0b00000, 0b00000, 0b00000, 0b11111, 0b01010, 0b10001, 0b00000, 0b00000, ]; const SYMBOL6: [u8; 8] = [ 0b00010, 0b00010, 0b00100, 0b11000, 0b10000, 0b01000, 0b10100, 0b00010, ]; }
Declare them as character
#![allow(unused)] fn main() { lcd.custom_char(&mut timer, &SYMBOL1, 0); lcd.custom_char(&mut timer, &SYMBOL2, 1); lcd.custom_char(&mut timer, &SYMBOL3, 2); lcd.custom_char(&mut timer, &SYMBOL4, 3); lcd.custom_char(&mut timer, &SYMBOL5, 4); lcd.custom_char(&mut timer, &SYMBOL6, 5); }
Display
Let’s write the first 3 grids into the first row, then the second half into the second row of the LCD display.
#![allow(unused)] fn main() { lcd.set_cursor(&mut timer, 0, 4) .write(&mut timer, CustomChar(0)) .write(&mut timer, CustomChar(1)) .write(&mut timer, CustomChar(2)); lcd.set_cursor(&mut timer, 1, 4) .write(&mut timer, CustomChar(3)) .write(&mut timer, CustomChar(4)) .write(&mut timer, CustomChar(5)); }
Clone the existing project
You can clone (or refer) project I created and navigate to the lcd-custom-multi
folder.
git clone https://github.com/ImplFerris/pico2-rp-projects
cd pico2-projects/lcd-custom-multi/