Blinking an External LED
In this section, we’ll use an external LED to blink.
You'll need some basic electronic components
Hardware Requirements
- LED
- Resistor
- Jumper wires
Refer the Raspberry pi guide for the hardware setup. I actually used it with breadboard instead.
Components Overview
-
LED: An LED (Light Emitting Diode) lights up when current flows through it. The longer leg (anode) connects to positive, and the shorter leg (cathode) connects to ground. We'll connect the anode to GP13 (with a resistor) and the cathode to GND.
-
Resistors: A resistor limits the current in a circuit to protect components like LEDs. Its value is measured in Ohms (Ω). We'll use a 330 ohm resistor to safely power the LED.
Pico Pin | Wire | Component |
---|---|---|
GPIO 13 |
|
Resistor |
Resistor |
|
Anode (long leg) of LED |
GND |
|
Cathode (short leg) of LED |
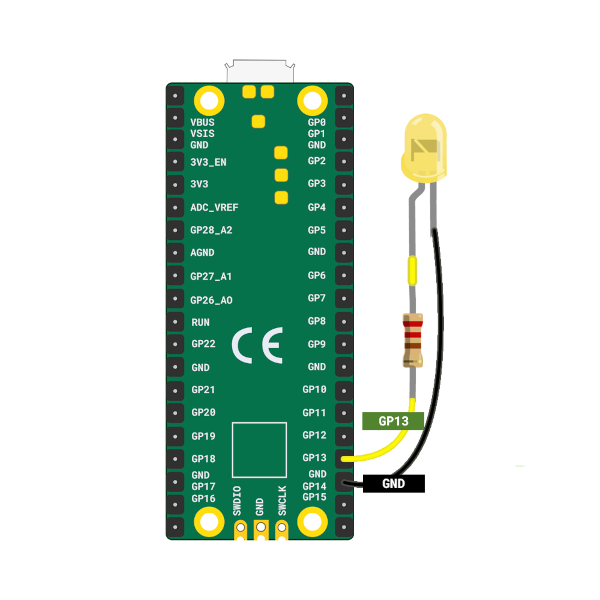
Code
There isn't much change in the code. We showcase how to blink an external LED connected to GPIO 13. The code uses a push-pull output configuration to control the LED state. By setting the pin high, the LED is turned on, and by setting it low, the LED is turned off. This process is repeated in an infinite loop with a delay of 200 milliseconds between each state change, allowing the LED to blink at a consistent rate.
#![allow(unused)] fn main() { let mut led_pin = pins.gpio13.into_push_pull_output(); loop { led_pin.set_high().unwrap(); delay.delay_ms(200); led_pin.set_low().unwrap(); delay.delay_ms(200); } }
Clone the existing project
You can clone the blinky project I created and navigate to the external-led
folder to run this version of the blink program:
git clone https://github.com/ImplFerris/pico2-rp-projects
cd pico2-projects/external-led
How to Run?
You refer the "Running The Program" section